Triggering ServiceNow Scheduled Jobs on Demand: A Developer's Guide
- nathanlee142
- Mar 21
- 4 min read
Updated: Mar 26
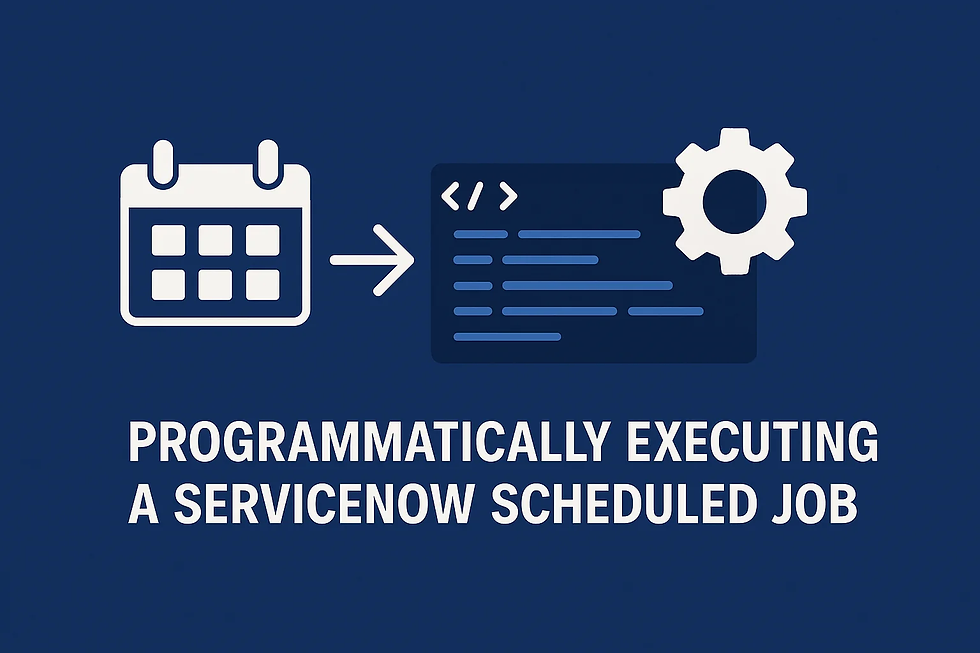
ServiceNow's Scheduled Jobs are a powerful way to automate routine tasks at specific times or intervals. However, there are scenarios where you might need to initiate these jobs immediately from a script or workflow, rather than waiting for their scheduled run time. This article will guide you through the process of programmatically executing a ServiceNow Scheduled Job on demand, providing you with the knowledge to enhance your automation capabilities.
Introduction: The Need for On-Demand Scheduled Job Execution in ServiceNow
While the scheduling functionality in ServiceNow is robust, sometimes you need more immediate control over when a job runs. For instance, after a large data import, you might want to immediately trigger a scheduled job that processes the imported data. Or, a specific user action in a workflow might necessitate the immediate execution of a maintenance job. Understanding how to trigger these jobs on demand from your scripts or workflows can significantly improve the responsiveness and efficiency of your ServiceNow applications.
Main Body: Programmatically Executing Scheduled Jobs in ServiceNow
ServiceNow provides a straightforward way to execute a Scheduled Job on demand using scripting. The primary method involves leveraging the SncTriggerSynchronizer class.
Using SncTriggerSynchronizer.executeNow()
The most direct approach to running a scheduled job on demand is by using the executeNow() method of the SncTriggerSynchronizer class. This method takes a GlideRecord object representing the scheduled job you want to execute.
Step-by-Step Instructions:
Identify the Scheduled Job: First, you need to know the sys_id of the Scheduled Job you want to trigger. You can find this by navigating to System Definition > Scheduled Jobs, locating the job, and copying its sys_id from the URL or the record information.
Retrieve the Scheduled Job Record: In your script (whether it's a Business Rule, Workflow Script, or another Scheduled Job), you'll need to retrieve the sys_trigger record (the table where Scheduled Jobs are stored) using the sys_id.
Execute the Job: Once you have the GlideRecord object for the Scheduled Job, you can pass it to the SncTriggerSynchronizer.executeNow() method.
Code Example:
// Get the sys_id of the scheduled job you want to run
var scheduledJobSysId = 'YOUR_SCHEDULED_JOB_SYS_ID'; // Replace with the actual sys_id
// Retrieve the scheduled job record
var scheduledJob = new GlideRecord('sys_trigger');
if (scheduledJob.get(scheduledJobSysId)) {
// Execute the scheduled job immediately
SncTriggerSynchronizer.executeNow(scheduledJob);
gs.log('Scheduled Job with sys_id ' + scheduledJobSysId + ' has been triggered on demand.');
} else {
gs.logError('Scheduled Job with sys_id ' + scheduledJobSysId + ' not found.');
}
Explanation:
We first declare a variable scheduledJobSysId and assign the sys_id of the target Scheduled Job to it. Remember to replace 'YOUR_SCHEDULED_JOB_SYS_ID' with the actual sys_id of your job.
We then create a new GlideRecord object for the sys_trigger table.
The get() method is used to retrieve the specific Scheduled Job record based on its sys_id.
If the record is found, the SncTriggerSynchronizer.executeNow(scheduledJob) line triggers the job to run immediately.
Appropriate log messages are included for informational and error handling purposes.
Note: The SncTriggerSynchronizer.executeNow() method only works with Scheduled Script Executions (type = "script") and not with other types of scheduled jobs like reports or events. Make sure the target Scheduled Job uses a script block.
Practical Use Cases:
Post-Data Import Processing: After importing a large dataset, you can trigger a scheduled job to process or validate the new data.
Workflow Integration: A workflow might reach a stage where a specific scheduled job needs to be executed before the workflow can proceed.
Manual Intervention: In certain situations, an administrator might need to manually trigger a scheduled job outside of its regular schedule via a script execution.
Alternative Solution: Moving Script Logic to a Script Include
Another approach, as suggested in the community discussion, is to move the core logic of your Scheduled Job into a Script Include. You can then call the function within the Script Include directly from your other scripts or workflows.
Pros:
Reusability: The logic can be easily called from multiple places without directly interacting with the scheduling mechanism.
Testability: Script Includes are generally easier to test in isolation.
Cons:
Not Truly Running the Scheduled Job: This approach bypasses the actual Scheduled Job record and its specific configurations (like run as user).
Potential Context Differences: The script might run under a different user context if called directly from another script.
Choose the approach that best fits your specific requirements. If you need to ensure the Scheduled Job runs with its defined configurations, using SncTriggerSynchronizer.executeNow() is the recommended method.
Conclusion: Empowering Your ServiceNow Automation
The ability to programmatically trigger Scheduled Jobs on demand in ServiceNow provides a significant level of flexibility in your automation efforts. By using the SncTriggerSynchronizer.executeNow() method, you can seamlessly integrate scheduled tasks into your scripts and workflows, ensuring timely execution when needed. Remember to carefully identify the Scheduled Job you want to trigger and use its GlideRecord object with the executeNow() method. This technique will empower you to build more responsive and efficient ServiceNow applications.