Handling "Price" Fields in ServiceNow Service Catalog: A Practical Guide
- kelly.ryu
- Mar 21
- 4 min read
Updated: Mar 26
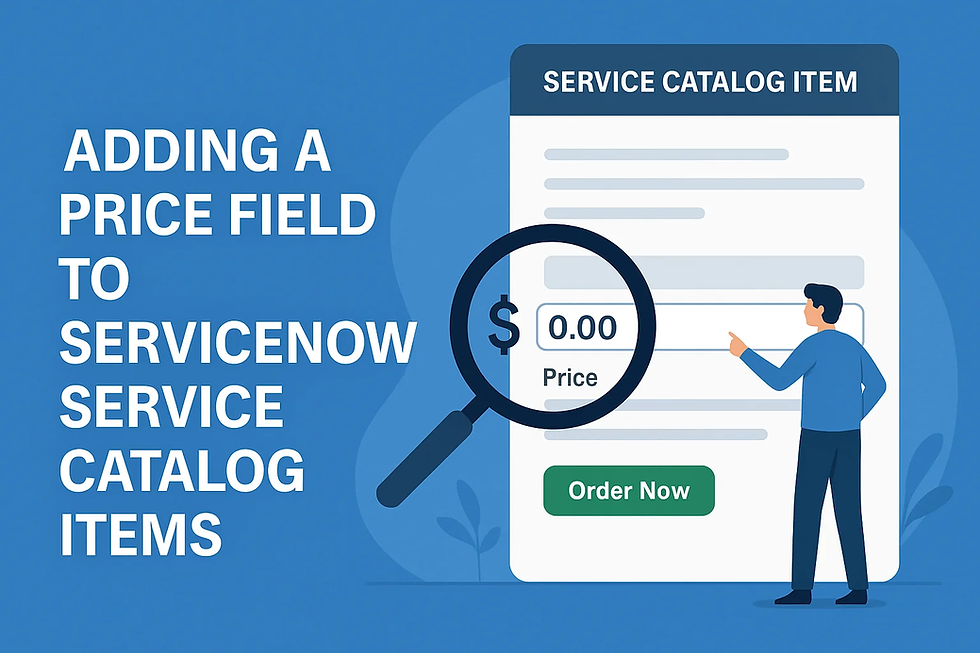
Are you looking to include a price or cost field within your ServiceNow Service Catalog items? While ServiceNow doesn't offer a dedicated "Price" variable type out-of-the-box, fear not! This article provides a workaround using a combination of scripting and readily available variable types to achieve the desired functionality. This is particularly useful when you need to display costs or allow users to input price-related information directly within the catalog item request. Let's explore how to implement this solution.
Understanding the Challenge and Available Solutions
The core challenge is the absence of a "Price" variable type within the Service Catalog. While a currency field type exists more broadly in ServiceNow, it's not available when creating variables for catalog items. This means we need to find creative solutions to validate and format user input to ensure it adheres to a specific currency format (e.g., $0.00).
Fortunately, there are a couple of methods you can follow:
Client-Side Scripting with Input Validation: This approach uses a Single Line Text variable combined with client-side scripting (onChange client script) to validate the user's input format. This ensures that the value entered conforms to the desired currency format before submission.
UI Script for Dynamic Formatting: This solution utilizes a UI Script that will format entered data to a currency string, such as $#,###.##.
Implementing Client-Side Validation for "Price" Fields
This method focuses on validating the user's input in real-time, providing immediate feedback if the entered value is not in the correct format. Here's a step-by-step guide:
Step 1: Create a Single Line Text Variable
In your Service Catalog item, create a new variable of type "Single Line Text." Name it appropriately, such as "Cost" or "Price."
Step 2: Write an onChange Client Script
Create a new "onChange" Client Script associated with your catalog item and the "Cost" variable. This script will trigger whenever the user changes the value in the "Cost" field.
Step 3: Implement the Validation Logic
Paste the following script into your client script. This script performs several checks:
Ensures the first character is a dollar sign ($).
Verifies that characters after the dollar sign are numeric.
Confirms the presence of a decimal point.
Enforces that there are exactly two digits after the decimal point.
function onChange(control, oldValue, newValue, isLoading) {
if (isLoading || newValue == '') {
return;
}
var cost = g_form.getValue('cost');
cost = cost.trim();
var firstChar = cost.substring(0,1);
if (firstChar != '$') {
alert ("Please enter cost in $0.00 format");
g_form.setValue("cost", oldValue);
return;
}
var costType = isNaN(cost.substring(1));
if (costType == true) {
alert ("Please enter cost in $0.00 format");
g_form.setValue("cost", oldValue);
return;
}
var num = cost.substring(1);
if (num.indexOf('.') == -1) {
alert ("Please enter cost in $0.00 format");
g_form.setValue("cost", oldValue);
return;
}
var decNum = num.substring(num.indexOf('.')+1, num.length);
if (decNum.length != 2) {
alert ("Please enter cost in $0.00 format");
g_form.setValue("cost", oldValue);
return;
}
}
Step 4: Customize and Enhance (Optional)
Error Message Customization: Modify the alert messages to provide more specific instructions to the user.
Allowing Commas: If you need to support commas in the input (e.g., $1,000.00), adjust the isNaN check.
Implementing UI Script for Dynamic Formatting
This method focuses on formatting the user's input on the fly, to ensure it's displayed correctly. Here's a step-by-step guide:
Step 1: Create a Single Line Text Variable
In your Service Catalog item, create a new variable of type "Single Line Text." Name it appropriately, such as "Cost" or "Price."
Step 2: Create a Global UI Script
Create a new Global UI Script. Name it appropriately, such as "FormatPrice".
Step 3: Implement the Formatting Logic
Paste the following script into your client script. This script formats the entered string data to $#,###.##
function FormatPrice(varName, strValue) {
//varName is the variable name
//strValue is the entered value of the Single Line Text variable
var xNum = Number(strValue.replace(/[^0-9.]/g, "")).toFixed(2);
var xStr = xNum.toString();
xStr = '$' + xStr.replace(/\B(?=(\d{3})+(?!\d))/g, ",");
g_form.setValue(varName, xStr);
return;
}
Step 4: Create an onChange Client Script
Create a new "onChange" Client Script associated with your catalog item and the "Cost" variable. This script will trigger whenever the user changes the value in the "Cost" field.
Step 5: Call the UI Script function to format the data
Paste the following script into your client script.
function onChange(control, oldValue, newValue, isLoading) {
if (isLoading || newValue == '') {
return;
}
FormatPrice('cost', newValue);
return;
}
Step 6: Customize and Enhance (Optional)
Support different currencies: Modify the xStr variable to support different currencies.
Tailoring ServiceNow to Your Needs
While ServiceNow's Service Catalog may not have a dedicated "Price" variable type, you can easily work around this limitation using scripting. By implementing client-side validation or UI scripts, you can effectively manage and format price or cost-related information within your catalog items. Remember to choose the solution that best fits your specific requirements and user experience goals. Now you can confidently handle price-related data in your ServiceNow Service Catalog.